Jason, that's brilliant -- thanks!
I've modified your script slightly to hide the username field and append a randomly generated number to the username (to reduce the chances of a user registering with a conflicting username; as noted in the code comments, this isn't a fool-proof solution.) I also hid some other elements that I didn't want to show on the registration page.
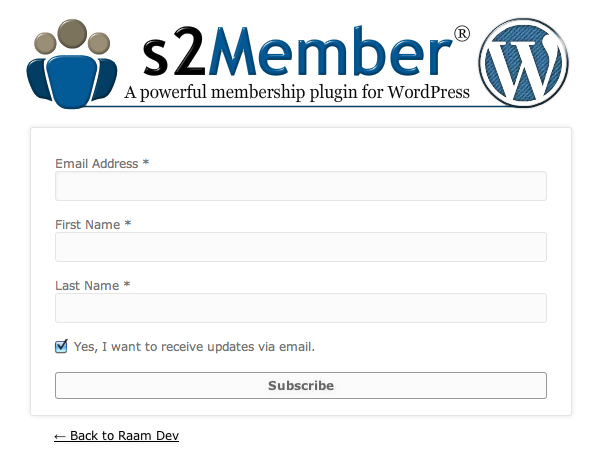
For those looking to create a registration form with the username field hidden and the username automatically generated, here you go:
Create this directory and file:/wp-content/mu-plugins/email-to-username.php
- Code: Select all
<?php
add_action ("login_head", "s2_customize_login", 1000);
function s2_customize_login ()
{
?>
<script type = "text/javascript">
function getParameterByName(name)
{
name = name.replace(/[\[]/, "\\\[").replace(/[\]]/, "\\\]");
var regexS = "[\\?&]" + name + "=([^&#]*)";
var regex = new RegExp(regexS);
var results = regex.exec(window.location.href);
if(results == null)
return "";
else
return decodeURIComponent(results[1].replace(/\+/g, " "));
}
(function ($) /* Wraps this `$ = jQuery` routine. */
{
$.fn.swapWith = function (to) /* Utility extension for jQuery. */
{
return this.each (function ()
{
var $to = $ (to).clone (true), $from = $ (this).clone (true);
$(to).replaceWith ($from), $ (this).replaceWith ($to);
});
};
/**/
$(document).ready (function () /* Handles email-to-username on keyup. */
{
/* If this is the register page, customize it */
if(getParameterByName('action') == 'register'){
/* Generate random number to append to username,
hopefully making it unique (yes, this isn't perfect!) */
var randomNum = Math.ceil(Math.random()*999);
var email = 'input#user_email';
var login = 'input#user_login';
var firstname = 'input#ws-plugin--s2member-custom-reg-field-first-name';
var lastname = 'input#ws-plugin--s2member-custom-reg-field-last-name';
$('p.message').hide();
$('div.ws-plugin--s2member-custom-reg-field-divider-section').hide();
$('#reg_passmail').hide();
$('#nav').hide();
$('#wp-submit').val('Subscribe');
$(login).closest ('p').hide();
$(email).closest ('p').swapWith ($ (firstname).closest ('p'));
$(email).closest ('p').swapWith ($ (lastname).closest ('p'));
$(firstname).focus();
$(email).attr('tabindex', 50);
/* Fill hidden username field with first part of email address
and append randomNum to hopefully make it unique. */
$ (email).keyup (function ()
{
$(login).val ($.trim ($ (email).val ().split (/@/)[0].replace (/[^\w]/gi, '')) + randomNum.toString());
});
}
});
}) (jQuery);
</script>
<?php
}
?>
(This is based on Jason's version here:
viewtopic.php?f=36&t=14806&p=33812#p33812)
Note that I also hid a few other elements on the page. You can delete those lines if you don't want to hide those elements.